When the Android platform was introduced by Google, Android edittext was one of the basic UI widgets. It is used by Android developers to take inputs from the user. And, while typing the text, it usually hides the hint that may create a problem for identifying Edittext without a label. Then, Google launched an Android design support library that consists of snackbar, floating action button, floating label in Android for edittext, and floating edittext Android.
This Android floating label for edittext is the concept of showing a label as a hint when the user is entering information in the edittext. When the user taps on the edittext that hint moves on the top as a floating hint edittext Android. In this Android app tutorial, we are going to learn how to add this floating label Android for edittext.
Table of Contents
Steps to Add Floating Label Android for Edittext
Open your Android Studio and create a new project.
In the next tab, select your target Android device.
Select Base Activity and click on next.
Lastly, customize the activity.
Adding Dependency
To display floating label in EditText control we need to add below dependencies.
dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', { exclude group: 'com.android.support', module: 'support-annotations' }) compile 'com.android.support:appcompat-v7:25.1.1' compile 'com.android.support:design:25.1.1' testCompile 'junit:junit:4.12' }
Want to Create an Android Application?
Get started on creating your own Android app with our expert app development services.
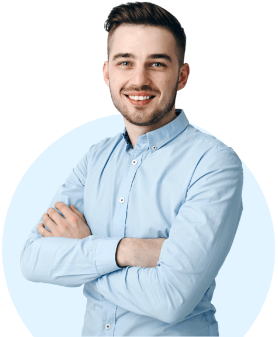
Now, Add EditText and TextInputLayout.
res/layout/content_main.xml
Add EditText inside TextInputLayout which you can find in android.support.design.widget.TextInputLayout
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/content_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:orientation="vertical" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="com.spaceo.textinputlayoutdemo.MainActivity" tools:showIn="@layout/activity_main"> <android.support.design.widget.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <EditText android:id="@+id/edFirstName" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:hint="@string/hint_first_name" android:inputType="textCapWords"/> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"/> <EditText android:id="@+id/edLastName" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:hint="@string/hint_last_name" android:inputType="textCapWords"/> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <EditText android:id="@+id/edEmailAdress" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:hint="Email Address" android:inputType="textEmailAddress"/> </android.support.design.widget.TextInputLayout> <android.support.design.widget.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="20dp"> <EditText android:id="@+id/edPhone" android:layout_width="match_parent" android:layout_height="wrap_content" android:ems="10" android:hint="Phone Number" android:inputType="textEmailAddress"/> </android.support.design.widget.TextInputLayout> <Button android:layout_width="match_parent" android:layout_marginTop="20dp" android:text="Submit" android:textAllCaps="false" android:layout_height="wrap_content"/> </LinearLayout>
That’s it!
Now, with the knowledge of how to add floating labels in Android apps, you can create looking forms and login pages. In fact, you can further experiment with different available attributes of the Android design support library. Below, we have tried to answer a few of the most common questions that we have been receiving from our readers:
FAQs
What is the Android design support library?
The Design Support Library enables developers to build upon material design components like navigation drawers, snackbars, and tabs.
How to set text in an EditText?
You can set Text in an EditText field by adding the following code:
editText.setText("Hello world!"); editText.setText(R.string.hello_world);
How can I reset the error state of the EditText?
You can use the following code to do that:
inputLayoutName.setErrorEnabled(false); inputLayoutEmail.setErrorEnabled(false); inputLayoutPassword.setErrorEnabled(false); inputLayoutName.setError(null); inputEmail.setError(null); inputPassword.setError(null);
How to change the color of the floating label?
You can change the color of the floating label from colors.xml.
Conclusion
We hope this tutorial will help you add a floating label for Edittext using the design support library. If you’re implementing the Android design support library attributes into your startup or business app, then it’s better that you consult with an expert first. The implementation might seem easy, but it’s advisable to consult with either an expert or a leading Android app development company to make sure that it has done right.